If you are here because you want to change the way the site looks to new browsers then you may want to take a look at the
Initial Settings feature in Tools | Options
. You do not normally need the Custom Content plugin to configure
the defaults for the web site.
The documentation for the Initial Settings feature can be found here.
How to change settings
The web site created by Virtual Radar Server is composed of a small number of HTML files and a large number of JavaScript files. The JavaScript is stored in <Program Files>\VirtualRadar\Web\Script.
Don't change those files, it won't work. The server will not serve modified files from that folder. You will have to reinstall Virtual Radar Server to get the original versions of those files back.
Most of the JavaScript stores its default settings in an object called VRS.globalOptions. You can inject content into the site to change these options so they're more to your liking.
You can also use the same technique to add to the site's CSS.
Scripts that change the settings or add CSS carry a small risk of breaking with future versions of Virtual Radar Server but that risk is very small. They are the most future-proof kind of alteration that you can make to the site.
The Template
Customising VRS.globalOptions involves injecting some JavaScript into the Desktop.html and Mobile.html pages. Create a file called CustomGlobalOptions.html on your computer (the name is not important, you can call it anything) and copy the following into it:
<script type="text/javascript"> if(VRS && VRS.globalDispatch && VRS.serverConfig) { VRS.globalDispatch.hook(VRS.globalEvent.bootstrapCreated, function(bootStrap) { // This will run once, just after the server configuration has been loaded // but before any of the JavaScript objects are initialised. // ADD YOUR CONTENT AFTER THIS LINE }); } </script>
In the Custom Content plugin options configure the plugin to inject your file at the END of the HEAD for every HTML file (an address of *):
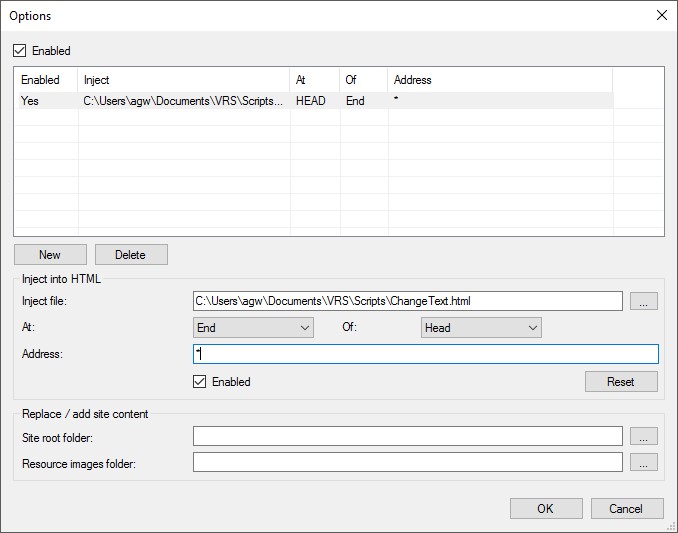
You don't need to restart the server - just reload the web page and the content of your HTML file will be injected into every HTML page served by the site. The file that contains the content is reloaded every time the user requests the web page that it has been injected into, so you do not need to do anything special to get changes picked up. You just need to reload the web page.
A Test Example
This script just displays an alert box with the text 'It worked!' when the page is loaded. If you use this script and you don't get the message then there's something wrong somewhere.
<script type="text/javascript"> if(VRS && VRS.globalDispatch && VRS.serverConfig) { VRS.globalDispatch.hook(VRS.globalEvent.bootstrapCreated, function(bootStrap) { alert('It worked!'); }); } </script>
Example Scripts
This script turns off altitude stalks by default and hides the options that would allow the user to switch them on:
<script type="text/javascript"> if(VRS && VRS.globalDispatch && VRS.serverConfig) { VRS.globalDispatch.hook(VRS.globalEvent.bootstrapCreated, function(bootStrap) { VRS.globalOptions.aircraftMarkerAllowAltitudeStalk = false; }); } </script>
This script disables trails for all aircraft and hides the options that would allow the user to switch them on:
<script type="text/javascript"> if(VRS && VRS.globalDispatch && VRS.serverConfig) { VRS.globalDispatch.hook(VRS.globalEvent.bootstrapCreated, function(bootStrap) { VRS.globalOptions.suppressTrails = true; }); } </script>
This script forces the refresh interval to three seconds (3000 ms) and stops the user from changing it:
<script type="text/javascript"> if(VRS && VRS.globalDispatch && VRS.serverConfig) { VRS.globalDispatch.hook(VRS.globalEvent.bootstrapCreated, function(bootStrap) { VRS.globalOptions.aircraftListFixedRefreshInterval = 3000; }); } </script>
This script sets four lines of marker text - registration, flight level, callsign and route:
<script type="text/javascript"> if(VRS && VRS.globalDispatch && VRS.serverConfig) { VRS.globalDispatch.hook(VRS.globalEvent.bootstrapCreated, function(bootStrap) { VRS.globalOptions.aircraftMarkerPinTextLines = 4; VRS.globalOptions.aircraftMarkerDefaultPinTexts = [ VRS.RenderProperty.Registration, VRS.RenderProperty.FlightLevel, VRS.RenderProperty.Callsign, VRS.RenderProperty.RouteShort ]; }); } </script>
By default callsigns that might be incorrect are shown with an asterisk against them. This script stops them from being shown altogether so that only callsigns that are definitely correct are shown:
<script type="text/javascript"> if(VRS && VRS.globalDispatch && VRS.serverConfig) { VRS.globalDispatch.hook(VRS.globalEvent.bootstrapCreated, function(bootStrap) { VRS.globalOptions.aircraftHideUncertainCallsigns = true; }); } </script>
This addition to the standard CSS adds a highlight when the mouse hovers over an unselected row in the aircraft list.
<style media="screen" type="text/css"> .aircraftList .vrsEven:hover, .aircraftList .vrsOdd:hover { color: #ffffff; background-color: #327CD8; } </style>
VRS.globalOptions Settings
Some of the options refer to enum values - these can be found in the enums.ts / enums.js source:
https://github.com/vradarserver/vrs/blob/master/VirtualRadar.WebSite/Site/Web/script/vrs/enums.js
compiled from
https://github.com/vradarserver/vrs/blob/master/VirtualRadar.WebSite/Site/Web/script/vrs/enums.ts
Aircraft details
VRS.globalOption | Description |
---|---|
aircraftAutoSelectClosest | True if auto-select closest is enabled by default. |
aircraftAutoSelectEnabled | True if auto-select is enabled by default. |
aircraftAutoSelectFilters | The initial array of VRS.AircraftFilter objects that will be used by auto-select. |
aircraftAutoSelectFiltersLimit | The maximum number of auto-select filters that the user can enter. |
aircraftAutoSelectOffRadarAction | The VRS.OffRadarAction enum value describing the default auto-select behaviour when an aircraft goes off radar. |
aircraftHideUncertainCallsigns | If true then uncertain callsigns are never shown. |
aircraftInfoWindowAllowConfiguration | True if the user can configure the infowindow settings. |
aircraftInfoWindowClass | The CSS class to assign to info windows. |
aircraftInfoWindowDistinguishOnGround | True if aircraft on the ground should show an altitude of GND. |
aircraftInfoWindowEnabled | True if the info window is enabled by default. |
aircraftInfoWindowEnablePanning | True if the map should pan to the info window when it opens. |
aircraftInfoWindowFlagUncertainCallsigns | True if uncertain callsigns are to be flagged as such. |
aircraftInfoWindowItems | An array of VRS.RenderProperty values that are shown by default in the info window. |
aircraftInfoWindowShowUnits | True if units should be shown in the info window. |
aircraftMaxAvgSignalLevelHistory | The number of signal level values that the average signal level is calculated over. |
aircraftPictureSizeDesktopDetail | The width or height in pixels of aircraft pictures on the desktop page. |
aircraftPictureSizeInfoWindow | The width and height in pixels of the mobile page's info window. |
aircraftPictureSizeIPadDetail | The width or height in pixels of aircraft pictures when viewed on tablets. |
aircraftPictureSizeIPhoneDetail | The width or height in pixels of aircraft pictures when viewed on phones. |
aircraftPictureSizeList | The width and height in pixels of aircraft picture thumbnails in the aircraft list. |
airportDataApiThumbnailsUrl | The default URL for airportdata.com thumbnails JSON fetches. |
airportDataApiTimeout | The number of milliseconds to wait before timing out a fetch of airportdata.com JSON. |
detailPanelAirportDataThumbnails | The number of airportdata.com thumbnails to show. |
detailPanelDefaultItems | An array of VRS.RenderProperty values that are shown by default in the aircraft detail panel. |
detailPanelDefaultShowUnits | True if the aircraft details panel should default to showing distance / speed / height units. |
detailPanelDistinguishOnGround | True if altitudes should show 'GND' when the aircraft is on the ground. |
detailPanelFlagUncertainCallsigns | True if uncertain callsigns are to be flagged up on the detail panel. |
detailPanelShowAircraftLinks | True to show the links for an aircraft, false to suppress them. |
detailPanelShowCentreOnAircraft | True to show a link to centre the map on the selected aircraft. |
detailPanelShowEnableAutoSelect | True to show a link to enable and disable auto-select, false to suppress the link. |
detailPanelShowSeparateRouteLink | Show a separate link to add or correct routes if the detail panel is showing routes. |
detailPanelUserCanConfigureItems | True if the user can change the items shown for an aircraft in the details panel. |
detailPanelUseShortLabels | True if the short list heading labels should be used in the aircraft detail panel. |
linkClass | The CSS class to assign to links. |
linkSeparator | The string to display between links in groups of external links. |
suppressTrails | If true then trails are never shown for any aircraft. |
Aircraft lists
VRS.globalOption | Description |
---|---|
aircraftListDataType | The format that the aircraft list is returned in. |
aircraftListDefaultFiltersEnabled | True if filters are enabled by default. |
aircraftListDefaultSortOrder | (See Note 1) The default sort order of the aircraft list. |
aircraftListFilters | The initial array of VRS.AircraftFilter objects that the list is filtered by. |
aircraftListFiltersLimit | The maximum number of list filters that the user can enter. |
aircraftListFixedRefreshInterval | The number of milliseconds between refreshes, -1 if the user can configure this themselves. |
aircraftListFlightSimUrl | The URL that the aircraft list JSON is fetched from when running in Flight Simulator X mode. |
aircraftListHideAircraftNotOnMap | True if aircraft that are not on display are hidden from the list, false if they are not. |
aircraftListRequestFeedId | The ID of the feed to request. If undefined then the default feed configured on the server is fetched. |
aircraftListRetryInterval | The number of milliseconds to wait before fetching an aircraft list after a failure. |
aircraftListShowEmergencySquawks | Indicates the precedence given to aircraft transmitting emergency squawks in the aircraft list. |
aircraftListShowInteresting | Indicates the precedence given to aircraft flagged as interesting in the aircraft list. |
aircraftListTimeout | The number of milliseconds before an aircraft list fetch will time out. |
aircraftListUrl | The URL that the aircraft list JSON is fetched from. |
aircraftListUserCanChangeFeeds | True if the user can change feeds, false if they cannot. |
listPluginDefaultColumns | An array of VRS.RenderProperty values that are shown by default in the aircraft list. |
listPluginDefaultShowUnits | True if units should be shown by default. |
listPluginDistinguishOnGround | True if altitudes should distinguish between a value of 0 and aircraft that are on the ground. |
listPluginFlagUncertainCallsigns | True if uncertain callsigns should be flagged in the aircraft list. |
listPluginShowHideAircraftNotOnMap | True if the link to hide aircraft not on map should be shown. |
listPluginShowPause | True if a pause link should be shown on the list, false if it should not. |
listPluginShowSorterOptions | True if sorter options should be shown on the list configuration panel. |
listPluginUserCanConfigureColumns | True if the user can configure the aircraft list columns. |
Map options
VRS.globalOption | Description |
---|---|
aircraftMarkerAllowAltitudeStalk | True if altitude stalks can be shown, false if they are permanently suppressed. |
aircraftMarkerAllowPinText | True to allow the user to display pin text on the markers. This can be overridden by server options. |
aircraftMarkerAllowRangeCircles | True if range circles are to be allowed, false if they are to be suppressed. |
aircraftMarkerAltitudeTrailHigh | The high range to use when colouring altitude trails, in feet. Altitudes above this are coloured red. |
aircraftMarkerAltitudeTrailLow | The low range to use when colouring altitude trails, in feet. Altitudes below this are coloured white. |
aircraftMarkerAlwaysPlotSelected | True to always plot the selected aircraft, even if it is not on the map. This preserves the selected aircraft's trail. |
aircraftMarkerClustererEnabled | True if clusters of aircraft should be presented as a single icon. |
aircraftMarkerClustererMaxZoom | The zoom level at which clusters of aircraft should be presented as a single icon. Smaller numbers are further away from the ground. |
aircraftMarkerClustererMinimumClusterSize | The number of aircraft that have to be grouped together before they become merged into a single cluster icon. |
aircraftMarkerClustererUserCanConfigure | True if the user can configure the parameters for aircraft marker clustering. |
aircraftMarkerDefaultPinTexts | (See Note 2) The initial array of VRS.RenderProperty values to show on the markers. |
aircraftMarkerHideEmptyPinTextLines | True if empty pin text lines are to be hidden. |
aircraftMarkerHideNonAircraftZoomLevel | The zoom level at which non-aircraft traffic is hidden. Lower numbers are further away from the ground. |
aircraftMarkerMaximumPinTextLines | The maximum number of lines of pin text that a user can show on markers. |
aircraftMarkerMovingMapOn | True if the moving map is switched on by default, false if it is not. |
aircraftMarkerOnlyUsePre22Icons | True if only the old pre-version 2.2 aircraft icon should be shown. |
aircraftMarkerPinTextLineHeight | The pixel height for each line of pin text on a marker. |
aircraftMarkerPinTextLines | The number of lines of pin text to show on markers. |
aircraftMarkerPinTextWidth | The pixel width for markers with pin text drawn on them. |
aircraftMarkerRangeCircleCount | The number of range circles to display around the current location. |
aircraftMarkerRangeCircleDistanceUnit | The VRS.Distance value indicating the units for aircraftMarkerRangeCircleInterval |
aircraftMarkerRangeCircleEvenColour | The CSS colour for the even range circles. |
aircraftMarkerRangeCircleEvenWeight | The width in pixels for the even range circles. |
aircraftMarkerRangeCircleInterval | The number of distance units between each successive range circle. |
aircraftMarkerRangeCircleMaxCircles | The maximum number of circles that the user can show. |
aircraftMarkerRangeCircleMaxInterval | The maximum interval that the user can request for a range circle. |
aircraftMarkerRangeCircleMaxWeight | The maximum weight that the user can specify for a range circle. |
aircraftMarkerRangeCircleOddColour | The CSS colour for the odd range circles. |
aircraftMarkerRangeCircleOddWeight | The width in pixels for the odd range circles. |
aircraftMarkerRotate | True if markers are allowed to rotate to indicate the direction of travel, assuming that the natural state points due north. |
aircraftMarkerRotationGranularity | The smallest number of degrees an aircraft has to rotate through before its marker is rotated. |
aircraftMarkers | (See Note 7) An array of the aircraft icons that can represent different types of aircraft on the map. |
aircraftMarkerShowAltitudeStalk | True if altitude stalks are shown by default, false if they are not. |
aircraftMarkerShowNonAircraftTrails | True if non-aircraft traffic should have trails drawn for them. |
aircraftMarkerShowRangeCircles | True if range circles are to be shown by default, false if they are not. |
aircraftMarkerShowTooltip | True to show tooltips on aircraft markers, false otherwise. |
aircraftMarkerSpeedTrailHigh | The high range to use when colouring speed trails, in knots. Speeds above this are coloured red. |
aircraftMarkerSpeedTrailLow | The low range to use when colouring speed trails, in knots. Speeds below this are coloured white. |
aircraftMarkerSuppressAltitudeStalkWhenZoomed | True to suppress the altitude stalk when zoomed out, false to always show it. |
aircraftMarkerSuppressAltitudeStalkZoomLevel | The map zoom level at which altitude stalks will be suppressed. |
aircraftMarkerSuppressTextOnImages | True if pin texts are rendered in JavaScript instead of at the server. Usually automatically set to true when server is running under Mono. |
aircraftMarkerTrailColourNormal | The CSS colour of trails for aircraft that are not selected. |
aircraftMarkerTrailColourSelected | The CSS colour of trails for aircraft that are selected. |
aircraftMarkerTrailDisplay | The VRS.TrailDisplay value that indicates which aircraft to display trails for. |
aircraftMarkerTrailType | The VRS.TrailType value that indicates the type of trail to display. |
aircraftMarkerTrailWidthNormal | The width in pixels of trails for aircraft that are not selected. |
aircraftMarkerTrailWidthSelected | The width in pixels of trails for aircraft that are selected. |
aircraftPositionMapClass | The CSS class to assign to the aircraft position map panel. |
aircraftRendererEnableDebugProperties | True if debug properties can be shown in aircraft lists, on markers etc. |
mapDraggable | True if the user can move the map. Overridden in some panels. |
mapGoogleMapHttpsUrl | The HTTPS URL to load Google Map JavaScript from. |
mapGoogleMapHttpUrl | The HTTP URL to load Google Map JavaScript from. |
mapGoogleMapTimeout | The number of milliseconds that the site will wait for before it times out the fetch of Google Maps JavaScript. |
mapGoogleMapUseHttps | True if the Google Maps JavaScript should be fetched over HTTPS. |
mapHighContrastMapStyle | The Google map styles to use for the high contrast map. See Google's map style wizard. |
mapLeafletNoWrap | True if Leaflet maps should have wrapping turned off. |
mapNextPageButtonClass | The CSS class to use on the next page button shown on the mobile map. |
mapNextPageButtonFilteredImage | The image to use for the "filtering is in effect" next page button. |
mapNextPageButtonFilteredSize | The size of the filtered next page button. |
mapNextPageButtonImage | The image to use for the normal next page button on the mobile map. |
mapNextPageButtonPausedImage | The image to use for paused next page on the mobile map. |
mapNextPageButtonPausedSize | The size of the paused next page button. |
mapNextPageButtonSize | The size of the normal next page button. |
mapScrollWheelActive | True if the scroll wheel zooms the map. Overridden in some panels. |
mapShowHighContrastStyle | True if the custom high contrast map style should be offered to the user. |
mapShowPointsOfInterest | True if maps should show points of interest by default. |
mapShowScaleControl | True if maps should show the scale control by default. |
mapShowStreetView | True if the StreetView icon should be shown on the map. |
menuClass | The CSS class to use on the menu. |
optionsDialogHeight | The height of the options dialog. |
optionsDialogModal | True if the options dialog is modal. |
optionsDialogPosition | The default position of the options dialog. |
optionsDialogWidth | The width of the options dialog. |
polarPlotAltitudeConfigs | (See Note 6) An array of objects that describe the colours and Z-index for each plot. The object is { low: number, high: number, colour: string, zIndex: number } where low and high describe the altitude range (-1 for an open end) and colour is a CSS string. |
polarPlotAutoRefreshSeconds | The number of seconds between the automatic refresh of all polar plots on display. Set to 0 to disable automatic refreshes. |
polarPlotDisplayOnStartup | An array of objects that describe the plots to show when the site starts. The object is { feedName: string, low: number, high: number } where low and high describe the altitude range (-1 for an open end) and feedName is the case insensitive name of a feed with a polar plotter attached. |
polarPlotEnabled | True if the user is allowed to see receiver range plots, false if they are to be suppressed. |
polarPlotFetchTimeout | The timeout in milliseconds when fetching polar plots from the server. |
polarPlotFetchUrl | The URL to fetch plotter JSON data from. |
polarPlotFillColourCallback | A function that is passed the feed ID, low altitude and high altitude and returns the CSS colour for the fill. |
polarPlotFillOpacity | The transparency of the polar plot fill. 0.0 is transparent, 1.0 is opaque. |
polarPlotStrokeColour | The CSS colour to use for the outline of the plot. If this is an empty string then the plot is outlined in the fill colour. |
polarPlotStrokeColourCallback | A function that is passed the feed ID, low altitude and high altitude and returns the CSS colour for the stroke. |
polarPlotStrokeOpacity | The transparency of the polar plot's outline. 0.0 is transparent, 1.0 is opaque. |
polarPlotStrokeWeight | The pixel width of the lines to draw around the edge of a polar plot. |
polarPlotUserConfigurable | True if the user can configure the receiver range plot colours and opacity. |
splitterBorderWidth | The width in pixels of the splitter bar. |
Miscellaneous
VRS.globalOption | Description |
---|---|
aircraftAllowRegistrationFlagOverride | True if you want to search for operator flags and silhouettes that match an aircraft's registration. Note that registrations can match the operator code or silhouette for other aircraft. |
aircraftBearingCompassSize | An object of { width: x, height: y } indicating the size of the compass bearing image. |
aircraftFlagUncertainCallsigns | True if callsigns that we're not 100% sure about are to be shown with an asterisk against them on. This overrides all other 'FlagUncertainCallsign' options. |
aircraftOperatorFlagSize | An object of { width: x, height: y } indicating the size of aircraft operator flag images. |
aircraftSilhouetteSize | An object of { width: x, height: y } indicating the size of aircraft silhouette images. |
aircraftTransponderTypeSize | An object of { width: x, height: y } indicating the size of the transponder type images. |
audioAnnounceOnlyAutoSelected | True if only auto-selected aircraft should have their details announced. |
audioAnnounceSelected | True if details of selected aircraft should be announced. |
audioDefaultVolume | The default volume for the audio control. Range is 0.0 to 1.0. |
audioEnabled | True if the audio features are enabled (can still be disabled on server). False if they are to be suppressed. |
audioTimeout | The number of milliseconds that must elapse before audio is timed-out. |
configSuppressEraseOldSiteConfig | True if the old site's configuration is not to be erased by the new site. If you set this to false it could lead the new site to be slighty less efficient when sending requests to the server. |
currentLocationConfigurable | True if the user is allowed to set their current location. |
currentLocationFixed | Set to an object of { lat: 1.234, lng: 5.678 }; to force the default current location (when the user has not assigned a location) to a fixed point rather than the server-configured initial location. |
currentLocationIconUrl | The URL of the marker to display on the map for the set current location marker. Set to null for the default Google Map marker. |
currentLocationImageSize | An object of { width: x, height: y } indicating the size of the current location marker in pixels. |
currentLocationImageUrl | The URL of the current location marker. |
currentLocationShowOnMap | True if the current location should be shown on the map. |
currentLocationUseBrowserLocation | (See Note 3) True if the browser location should be used as the current location. This overrides the map centre / user-supplied location options. |
currentLocationUseGeoLocation | True if the option to use the browser's current location should be shown. |
currentLocationUseMapCentreForFirstVisit | If true then on the first visit the user-supplied current location is set to the map centre. If false then the user must always choose a current location (i.e. the same behaviour as version 1 of the site). |
scriptManagerTimeout | The timeout in milliseconds when dynamically loading scripts (e.g. Google Maps and the language files). |
serverConfigDataType | The format that the server configuration will use. |
serverConfigIgnoreLanguage | True to ignore the language settings when importing the configuration stored on the server. |
serverConfigIgnoreRequestFeedId | True to ignore the feed ID to fetch when importing the configuration stored on the server. |
serverConfigIgnoreSplitters | True to ignore the splitter settings when importing the configuration stored on the server. |
serverConfigOverwrite | True to overwrite the existing configuration with the configuration stored on the server. |
serverConfigResetBeforeImport | True to erase the existing configuration before importing the configuration stored on the server. |
serverConfigRetryInterval | The number of milliseconds to wait before retrying a fetch of server configuration. |
serverConfigTimeout | The number of milliseconds to wait before timing out a fetch of server configuration. |
serverConfigUrl | The URL to fetch the server configuration from. |
unitDisplayAllowConfiguration | True if the user can configure the unit display options. |
unitDisplayAltitudeType | True if the altitude type should be shown. |
unitDisplayDistance | The default VRS.Distance unit for distances. |
unitDisplayFLHeightUnit | The VRS.Height unit that flight levels are displayed in. |
unitDisplayFLTransitionAltitude | The default flight level transition altitude. |
unitDisplayFLTransitionHeightUnit | The VRS.Height unit for unitDisplayFLTransitionAltitude. |
unitDisplayHeight | The default VRS.Height unit for altitudes. |
unitDisplayPressure | The default VRS.Pressure unit for air pressure. |
unitDisplaySpeed | The default VRS.Speed unit for speeds. |
unitDisplaySpeedType | True if the speed type should be shown. |
unitDisplayTrackType | True if the heading type should be shown. |
unitDisplayUsePressureAltitude | True to show pressure altitudes or false to show corrected altitudes by default. |
unitDisplayVerticalSpeedType | True if the vertical speed type should be shown. |
unitDisplayVsiPerSecond | (See Note 4) True if vertical speeds are to be shown per second rather than per minute. |
Reports
VRS.globalOption | Description |
---|---|
pagePanelClass | The CSS class to assign to page panels in the options dialog. |
reportDefaultPageSize | The default page size to show. Use -1 if you want to default to showing all rows. |
reportDefaultSortColumns | (See Note 5) The default sort order for reports. Note that the server will not accept any more than two sort columns. |
reportDefaultStepSize | The default step to show on the page size controls. |
reportDetailAddMapToDefaultColumns | (See Note 3) True if the map should be added to the default columns. |
reportDetailClass | The CSS class to assign to the report detail panel. |
reportDetailColumns | An array of VRS.ReportAircraftProperty and VRS.ReportFlightProperty values that are shown in the aircraft detail panel by default. |
reportDetailDefaultShowEmptyValues | True if empty values are to be shown. |
reportDetailDefaultShowUnits | True if the detail panel should show units by default. |
reportDetailDistinguishOnGround | True if the detail panel should show GND for aircraft that are on the ground. |
reportDetailShowAircraftLinks | True if external site links are to be shown. |
reportDetailShowSeparateRouteLink | True if the user should be shown a link to correct routes. |
reportDetailUserCanConfigureColumns | True if the user is allowed to configure which values are shown in the detail panel. |
reportFindAllPermutationsOfCallsign | True if all permutations of a callsign should be found. |
reportListClass | The CSS class to assign to the report list panel. |
reportListDefaultShowUnits | True if the default should be to show units. |
reportListDistinguishOnGround | True if aircraft on ground should be shown as an altitude of GND. |
reportListGroupBySortColumn | True if the report list should show group rows when the value of the first sort column changes. |
reportListGroupResetAlternateRows | True if the report list should reset the alternate row shading at the start of each group. |
reportListManyAircraftColumns | An array of VRS.ReportFlightProperty and VRS.ReportAircraftProperty values to show for reports with criteria that could cover many aircraft. |
reportListShowPagerBottom | True if the report list is to show a pager below the list. |
reportListShowPagerTop | True if the report list is to show a pager above the list. |
reportListSingleAircraftColumns | An array of VRS.ReportFlightProperty and VRS.ReportAircraftProperty values to show for reports on a single aircraft. |
reportListUserCanConfigureColumns | True if the user is allowed to configure the columns in the report list. |
reportMapClass | The CSS class to assign to the report map panel. |
reportMapScrollToAircraft | True if the map should automatically scroll to show the selected aircraft's path. |
reportMapShowPath | True if a line should be drawn between the start and end points of the aircraft's path. |
reportMapStartSelected | True if the start point should be displayed in the selected colours, false if the end point should show in selected colours. |
reportMaximumCriteria | The maximum number of criteria that can be passed to a report. |
reportPagerAllowPageSizeChange | True if the user can change the report page size, false if they cannot. |
reportPagerAllowShowAllRows | True if the user is allowed to show all rows simultaneously, false if they're not. |
reportPagerClass | The CSS class to assign to the report pager panel. |
reportPagerSpinnerPageSize | The number of pages to skip when paging up and down through the page number spinner. |
reportShowPermanentLinkToReport | True to show the permanent link to a report and its criteria. |
reportUrl | The URL to fetch report data from. |
reportUseRelativeDatesInLink | True to use relative dates in the permanent link. |
Note 1
The sort order is an array of objects with two properties, field (a VRS.AircraftListSortableField value - see enums.js) and ascending (true or false). The
number of elements in the array indicates how many fields the user can sort by. The default value is an array of three elements:
VRS.globalOptions.aircraftListDefaultSortOrder = [ { field: VRS.AircraftListSortableField.TimeTracked, ascending: true }, { field: VRS.AircraftListSortableField.None, ascending: true }, { field: VRS.AircraftListSortableField.None, ascending: true } ];
Note 2
The VRS.RenderProperty values are declared in enums.js. Note that not all VRS.RenderProperty values can be used as pin text (e.g. Picture and RouteFull cannot).
The default value is an array of three elements - if you declare fewer elements than there are in VRS.globalOptions.aircraftMarkerPinTextLines then the
missing elements are set to RenderProperty.None:
VRS.globalOptions.aircraftMarkerDefaultPinTexts = [ VRS.RenderProperty.Registration, VRS.RenderProperty.Callsign, VRS.RenderProperty.Altitude ];
Note 3
True if a mobile page is loaded, false if a desktop page is loaded. This is passed through the option VRS.globalOptions.isMobile which is set to true by the mobile pages.
Note 4
True if a flight simulator page is loaded, false if a desktop page is loaded. This is passed through the option VRS.globalOptions.isFlightSim which is set to true by the flight simulator pages.
Note 5
The report sort order is an array of objects with two properties, field (a VRS.ReportSortColumn value - see enums.js) and ascending (true or false). The
server will not sort by more than two fields, so it is pointless to set the array to more than two elements. The default report sort order is:
VRS.globalOptions.reportDefaultSortColumns = [ { field: VRS.ReportSortColumn.Date, ascending: true }, { field: VRS.ReportSortColumn.None, ascending: false } ];
Note 6
The altitude ranges in the array of colours and z-indexes for each polar plot (aka receiver range plot) must match the altitude ranges that
the server is tracking and sending for each polar plotter. These are not configurable, if you change the altitude ranges here then it will
not work. All you can do here is remove certain ranges entirely or change their colour / z-index. The default array is:
VRS.globalOptions.polarPlotAltitudeConfigs = VRS.globalOptions.polarPlotAltitudeConfigs || [ { low: -1, high: -1, colour: '#000000', zIndex: -5 }, { low: -1, high: 9999, colour: '#FFFFFF', zIndex: -1 }, { low: 10000, high: 19999, colour: '#00FF00', zIndex: -2 }, { low: 20000, high: 29999, colour: '#0000FF', zIndex: -3 }, { low: 30000, high: -1, colour: '#FF0000', zIndex: -4 } ];